Run a query
The first idea coming to mind when using a database is to execute queries. Let’s do it.
The following code will run a query straight in the current search page of BioloMICS. So open at least one Search Page, and try to view many records in its BioSheet.
Imports System
Imports System.Collections
Imports Biolomics.BioCallback
Imports Biolomics.SharedClasses
Public Class MyNewClass
' This function is called when running this script
Shared Sub Main()
' Create an instance of class MyNewClass and call its functions
Dim c As New MyNewClass
c.RunQuery()
End Sub
' ***** Run a query in the current SearchPage *****
Public Sub RunQuery()
Dim Where As XWhere = XWhere.GT(StaticFields.id, 2).And_(StaticFields.id, XWhere.OperationEnum.LT, 8).Or_(StaticFields.name, XWhere.OperationEnum.EndWith, "877")
Dim ErrorCode As Integer = Run.RunWhereQuery(Where)
If ErrorCode = 0 Then
Console.Write("No Error")
Else
DrawMessages()
End If
End Sub
' display all recent error messages
Public Sub DrawMessages()
Dim ErrorList As Generic.List(Of String) = Run.GetLastErrorMessage()
For Each errorMsg As String In Errorlist
Console.Write(vbcrlf & errorMsg)
Next
End Sub
End Class
This Query will select in the main BioloMICS window, all records with ID > 2 and ID < 8 or with a name finishing with “CBS 877”.
Compile and run it. Then go back to the main BioloMICS window and the BioSheet should contain only records whose names respect the query.
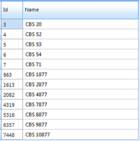
Any query can be processed this way.
Note that this query is only used to load records. Allowing a straight access to the database to all users would certainly lead to serious data damages. It is currently forbidden.
The way queries are generated is database independent.
For those who will run complex queries, here is the detailed analysis of the WHERE clause available from the XWhere class.